rawtypes.clang.cindex module¶
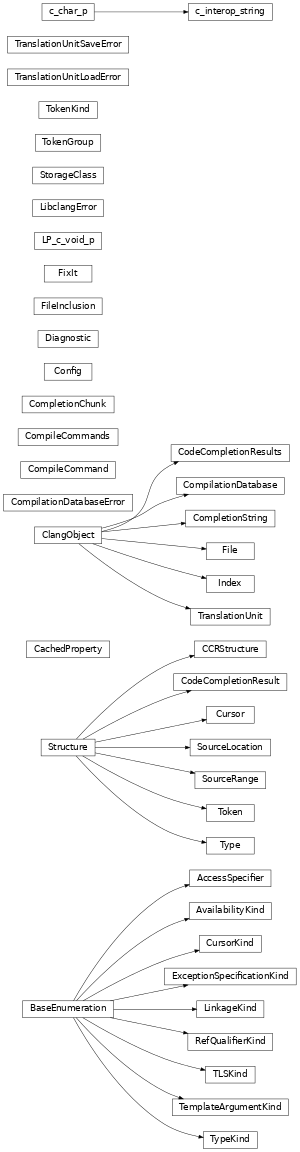
Clang Indexing Library Bindings¶
This module provides an interface to the Clang indexing library. It is a low-level interface to the indexing library which attempts to match the Clang API directly while also being "pythonic". Notable differences from the C API are:
string results are returned as Python strings, not CXString objects.
null cursors are translated to None.
access to child cursors is done via iteration, not visitation.
The major indexing objects are:
Index
The top-level object which manages some global library state.
TranslationUnit
High-level object encapsulating the AST for a single translation unit. These can be loaded from .ast files or parsed on the fly.
Cursor
Generic object for representing a node in the AST.
SourceRange, SourceLocation, and File
Objects representing information about the input source.
Most object information is exposed using properties, when the underlying API call is efficient.
- class rawtypes.clang.cindex.AvailabilityKind(value)¶
ベースクラス:
rawtypes.clang.cindex.BaseEnumeration
Describes the availability of an entity.
- AVAILABLE = AvailabilityKind.AVAILABLE¶
- DEPRECATED = AvailabilityKind.DEPRECATED¶
- NOT_ACCESSIBLE = AvailabilityKind.NOT_ACCESSIBLE¶
- NOT_AVAILABLE = AvailabilityKind.NOT_AVAILABLE¶
- class rawtypes.clang.cindex.Config¶
ベースクラス:
object
- library_path = None¶
- library_file = None¶
- compatibility_check = True¶
- loaded = False¶
- static set_library_path(path)¶
Set the path in which to search for libclang
- static set_library_file(filename)¶
Set the exact location of libclang
- static set_compatibility_check(check_status)¶
Perform compatibility check when loading libclang
The python bindings are only tested and evaluated with the version of libclang they are provided with. To ensure correct behavior a (limited) compatibility check is performed when loading the bindings. This check will throw an exception, as soon as it fails.
In case these bindings are used with an older version of libclang, parts that have been stable between releases may still work. Users of the python bindings can disable the compatibility check. This will cause the python bindings to load, even though they are written for a newer version of libclang. Failures now arise if unsupported or incompatible features are accessed. The user is required to test themselves if the features they are using are available and compatible between different libclang versions.
- lib¶
- get_filename()¶
- get_cindex_library()¶
- function_exists(name)¶
- class rawtypes.clang.cindex.CodeCompletionResults(ptr)¶
ベースクラス:
rawtypes.clang.cindex.ClangObject
- from_param()¶
- property results¶
- property diagnostics¶
- class rawtypes.clang.cindex.CompilationDatabase(obj)¶
ベースクラス:
rawtypes.clang.cindex.ClangObject
The CompilationDatabase is a wrapper class around clang::tooling::CompilationDatabase
It enables querying how a specific source file can be built.
- static from_result(res, fn, args)¶
- static fromDirectory(buildDir)¶
Builds a CompilationDatabase from the database found in buildDir
- getCompileCommands(filename)¶
Get an iterable object providing all the CompileCommands available to build filename. Returns None if filename is not found in the database.
- getAllCompileCommands()¶
Get an iterable object providing all the CompileCommands available from the database.
- class rawtypes.clang.cindex.CompileCommands(ccmds)¶
ベースクラス:
object
CompileCommands is an iterable object containing all CompileCommand that can be used for building a specific file.
- static from_result(res, fn, args)¶
- class rawtypes.clang.cindex.CompileCommand(cmd, ccmds)¶
ベースクラス:
object
Represents the compile command used to build a file
- property directory¶
Get the working directory for this CompileCommand
- property filename¶
Get the working filename for this CompileCommand
- property arguments¶
Get an iterable object providing each argument in the command line for the compiler invocation as a _CXString.
Invariant : the first argument is the compiler executable
- class rawtypes.clang.cindex.CursorKind(value)¶
ベースクラス:
rawtypes.clang.cindex.BaseEnumeration
A CursorKind describes the kind of entity that a cursor points to.
- static get_all_kinds()¶
Return all CursorKind enumeration instances.
- is_declaration()¶
Test if this is a declaration kind.
- is_reference()¶
Test if this is a reference kind.
- is_expression()¶
Test if this is an expression kind.
- is_statement()¶
Test if this is a statement kind.
- is_attribute()¶
Test if this is an attribute kind.
- is_invalid()¶
Test if this is an invalid kind.
- is_translation_unit()¶
Test if this is a translation unit kind.
- is_preprocessing()¶
Test if this is a preprocessing kind.
- is_unexposed()¶
Test if this is an unexposed kind.
- ADDR_LABEL_EXPR = CursorKind.ADDR_LABEL_EXPR¶
- ALIGNED_ATTR = CursorKind.ALIGNED_ATTR¶
- ANNOTATE_ATTR = CursorKind.ANNOTATE_ATTR¶
- ARRAY_SUBSCRIPT_EXPR = CursorKind.ARRAY_SUBSCRIPT_EXPR¶
- ASM_LABEL_ATTR = CursorKind.ASM_LABEL_ATTR¶
- ASM_STMT = CursorKind.ASM_STMT¶
- BINARY_OPERATOR = CursorKind.BINARY_OPERATOR¶
- BLOCK_EXPR = CursorKind.BLOCK_EXPR¶
- BREAK_STMT = CursorKind.BREAK_STMT¶
- CALL_EXPR = CursorKind.CALL_EXPR¶
- CASE_STMT = CursorKind.CASE_STMT¶
- CHARACTER_LITERAL = CursorKind.CHARACTER_LITERAL¶
- CLASS_DECL = CursorKind.CLASS_DECL¶
- CLASS_TEMPLATE = CursorKind.CLASS_TEMPLATE¶
- CLASS_TEMPLATE_PARTIAL_SPECIALIZATION = CursorKind.CLASS_TEMPLATE_PARTIAL_SPECIALIZATION¶
- COMPOUND_ASSIGNMENT_OPERATOR = CursorKind.COMPOUND_ASSIGNMENT_OPERATOR¶
- COMPOUND_LITERAL_EXPR = CursorKind.COMPOUND_LITERAL_EXPR¶
- COMPOUND_STMT = CursorKind.COMPOUND_STMT¶
- CONDITIONAL_OPERATOR = CursorKind.CONDITIONAL_OPERATOR¶
- CONSTRUCTOR = CursorKind.CONSTRUCTOR¶
- CONST_ATTR = CursorKind.CONST_ATTR¶
- CONTINUE_STMT = CursorKind.CONTINUE_STMT¶
- CONVERGENT_ATTR = CursorKind.CONVERGENT_ATTR¶
- CONVERSION_FUNCTION = CursorKind.CONVERSION_FUNCTION¶
- CSTYLE_CAST_EXPR = CursorKind.CSTYLE_CAST_EXPR¶
- CUDACONSTANT_ATTR = CursorKind.CUDACONSTANT_ATTR¶
- CUDADEVICE_ATTR = CursorKind.CUDADEVICE_ATTR¶
- CUDAGLOBAL_ATTR = CursorKind.CUDAGLOBAL_ATTR¶
- CUDAHOST_ATTR = CursorKind.CUDAHOST_ATTR¶
- CUDASHARED_ATTR = CursorKind.CUDASHARED_ATTR¶
- CXX_ACCESS_SPEC_DECL = CursorKind.CXX_ACCESS_SPEC_DECL¶
- CXX_BASE_SPECIFIER = CursorKind.CXX_BASE_SPECIFIER¶
- CXX_BOOL_LITERAL_EXPR = CursorKind.CXX_BOOL_LITERAL_EXPR¶
- CXX_CATCH_STMT = CursorKind.CXX_CATCH_STMT¶
- CXX_CONST_CAST_EXPR = CursorKind.CXX_CONST_CAST_EXPR¶
- CXX_DELETE_EXPR = CursorKind.CXX_DELETE_EXPR¶
- CXX_DYNAMIC_CAST_EXPR = CursorKind.CXX_DYNAMIC_CAST_EXPR¶
- CXX_FINAL_ATTR = CursorKind.CXX_FINAL_ATTR¶
- CXX_FOR_RANGE_STMT = CursorKind.CXX_FOR_RANGE_STMT¶
- CXX_FUNCTIONAL_CAST_EXPR = CursorKind.CXX_FUNCTIONAL_CAST_EXPR¶
- CXX_METHOD = CursorKind.CXX_METHOD¶
- CXX_NEW_EXPR = CursorKind.CXX_NEW_EXPR¶
- CXX_NULL_PTR_LITERAL_EXPR = CursorKind.CXX_NULL_PTR_LITERAL_EXPR¶
- CXX_OVERRIDE_ATTR = CursorKind.CXX_OVERRIDE_ATTR¶
- CXX_REINTERPRET_CAST_EXPR = CursorKind.CXX_REINTERPRET_CAST_EXPR¶
- CXX_STATIC_CAST_EXPR = CursorKind.CXX_STATIC_CAST_EXPR¶
- CXX_THIS_EXPR = CursorKind.CXX_THIS_EXPR¶
- CXX_THROW_EXPR = CursorKind.CXX_THROW_EXPR¶
- CXX_TRY_STMT = CursorKind.CXX_TRY_STMT¶
- CXX_TYPEID_EXPR = CursorKind.CXX_TYPEID_EXPR¶
- CXX_UNARY_EXPR = CursorKind.CXX_UNARY_EXPR¶
- DECL_REF_EXPR = CursorKind.DECL_REF_EXPR¶
- DECL_STMT = CursorKind.DECL_STMT¶
- DEFAULT_STMT = CursorKind.DEFAULT_STMT¶
- DESTRUCTOR = CursorKind.DESTRUCTOR¶
- DLLEXPORT_ATTR = CursorKind.DLLEXPORT_ATTR¶
- DLLIMPORT_ATTR = CursorKind.DLLIMPORT_ATTR¶
- DO_STMT = CursorKind.DO_STMT¶
- ENUM_CONSTANT_DECL = CursorKind.ENUM_CONSTANT_DECL¶
- ENUM_DECL = CursorKind.ENUM_DECL¶
- FIELD_DECL = CursorKind.FIELD_DECL¶
- FLOATING_LITERAL = CursorKind.FLOATING_LITERAL¶
- FOR_STMT = CursorKind.FOR_STMT¶
- FRIEND_DECL = CursorKind.FRIEND_DECL¶
- FUNCTION_DECL = CursorKind.FUNCTION_DECL¶
- FUNCTION_TEMPLATE = CursorKind.FUNCTION_TEMPLATE¶
- GENERIC_SELECTION_EXPR = CursorKind.GENERIC_SELECTION_EXPR¶
- GNU_NULL_EXPR = CursorKind.GNU_NULL_EXPR¶
- GOTO_STMT = CursorKind.GOTO_STMT¶
- IB_ACTION_ATTR = CursorKind.IB_ACTION_ATTR¶
- IB_OUTLET_ATTR = CursorKind.IB_OUTLET_ATTR¶
- IB_OUTLET_COLLECTION_ATTR = CursorKind.IB_OUTLET_COLLECTION_ATTR¶
- IF_STMT = CursorKind.IF_STMT¶
- IMAGINARY_LITERAL = CursorKind.IMAGINARY_LITERAL¶
- INCLUSION_DIRECTIVE = CursorKind.INCLUSION_DIRECTIVE¶
- INDIRECT_GOTO_STMT = CursorKind.INDIRECT_GOTO_STMT¶
- INIT_LIST_EXPR = CursorKind.INIT_LIST_EXPR¶
- INTEGER_LITERAL = CursorKind.INTEGER_LITERAL¶
- INVALID_CODE = CursorKind.INVALID_CODE¶
- INVALID_FILE = CursorKind.INVALID_FILE¶
- LABEL_REF = CursorKind.LABEL_REF¶
- LABEL_STMT = CursorKind.LABEL_STMT¶
- LAMBDA_EXPR = CursorKind.LAMBDA_EXPR¶
- LINKAGE_SPEC = CursorKind.LINKAGE_SPEC¶
- MACRO_DEFINITION = CursorKind.MACRO_DEFINITION¶
- MACRO_INSTANTIATION = CursorKind.MACRO_INSTANTIATION¶
- MEMBER_REF = CursorKind.MEMBER_REF¶
- MEMBER_REF_EXPR = CursorKind.MEMBER_REF_EXPR¶
- MODULE_IMPORT_DECL = CursorKind.MODULE_IMPORT_DECL¶
- MS_ASM_STMT = CursorKind.MS_ASM_STMT¶
- NAMESPACE = CursorKind.NAMESPACE¶
- NAMESPACE_ALIAS = CursorKind.NAMESPACE_ALIAS¶
- NAMESPACE_REF = CursorKind.NAMESPACE_REF¶
- NODUPLICATE_ATTR = CursorKind.NODUPLICATE_ATTR¶
- NOT_IMPLEMENTED = CursorKind.NOT_IMPLEMENTED¶
- NO_DECL_FOUND = CursorKind.NO_DECL_FOUND¶
- NULL_STMT = CursorKind.NULL_STMT¶
- OBJC_AT_CATCH_STMT = CursorKind.OBJC_AT_CATCH_STMT¶
- OBJC_AT_FINALLY_STMT = CursorKind.OBJC_AT_FINALLY_STMT¶
- OBJC_AT_SYNCHRONIZED_STMT = CursorKind.OBJC_AT_SYNCHRONIZED_STMT¶
- OBJC_AT_THROW_STMT = CursorKind.OBJC_AT_THROW_STMT¶
- OBJC_AT_TRY_STMT = CursorKind.OBJC_AT_TRY_STMT¶
- OBJC_AUTORELEASE_POOL_STMT = CursorKind.OBJC_AUTORELEASE_POOL_STMT¶
- OBJC_AVAILABILITY_CHECK_EXPR = CursorKind.OBJC_AVAILABILITY_CHECK_EXPR¶
- OBJC_BRIDGE_CAST_EXPR = CursorKind.OBJC_BRIDGE_CAST_EXPR¶
- OBJC_CATEGORY_DECL = CursorKind.OBJC_CATEGORY_DECL¶
- OBJC_CATEGORY_IMPL_DECL = CursorKind.OBJC_CATEGORY_IMPL_DECL¶
- OBJC_CLASS_METHOD_DECL = CursorKind.OBJC_CLASS_METHOD_DECL¶
- OBJC_CLASS_REF = CursorKind.OBJC_CLASS_REF¶
- OBJC_DYNAMIC_DECL = CursorKind.OBJC_DYNAMIC_DECL¶
- OBJC_ENCODE_EXPR = CursorKind.OBJC_ENCODE_EXPR¶
- OBJC_FOR_COLLECTION_STMT = CursorKind.OBJC_FOR_COLLECTION_STMT¶
- OBJC_IMPLEMENTATION_DECL = CursorKind.OBJC_IMPLEMENTATION_DECL¶
- OBJC_INSTANCE_METHOD_DECL = CursorKind.OBJC_INSTANCE_METHOD_DECL¶
- OBJC_INTERFACE_DECL = CursorKind.OBJC_INTERFACE_DECL¶
- OBJC_IVAR_DECL = CursorKind.OBJC_IVAR_DECL¶
- OBJC_MESSAGE_EXPR = CursorKind.OBJC_MESSAGE_EXPR¶
- OBJC_PROPERTY_DECL = CursorKind.OBJC_PROPERTY_DECL¶
- OBJC_PROTOCOL_DECL = CursorKind.OBJC_PROTOCOL_DECL¶
- OBJC_PROTOCOL_EXPR = CursorKind.OBJC_PROTOCOL_EXPR¶
- OBJC_PROTOCOL_REF = CursorKind.OBJC_PROTOCOL_REF¶
- OBJC_SELECTOR_EXPR = CursorKind.OBJC_SELECTOR_EXPR¶
- OBJC_STRING_LITERAL = CursorKind.OBJC_STRING_LITERAL¶
- OBJC_SUPER_CLASS_REF = CursorKind.OBJC_SUPER_CLASS_REF¶
- OBJC_SYNTHESIZE_DECL = CursorKind.OBJC_SYNTHESIZE_DECL¶
- OBJ_BOOL_LITERAL_EXPR = CursorKind.OBJ_BOOL_LITERAL_EXPR¶
- OBJ_SELF_EXPR = CursorKind.OBJ_SELF_EXPR¶
- OMP_ARRAY_SECTION_EXPR = CursorKind.OMP_ARRAY_SECTION_EXPR¶
- OMP_ATOMIC_DIRECTIVE = CursorKind.OMP_ATOMIC_DIRECTIVE¶
- OMP_BARRIER_DIRECTIVE = CursorKind.OMP_BARRIER_DIRECTIVE¶
- OMP_CANCELLATION_POINT_DIRECTIVE = CursorKind.OMP_CANCELLATION_POINT_DIRECTIVE¶
- OMP_CANCEL_DIRECTIVE = CursorKind.OMP_CANCEL_DIRECTIVE¶
- OMP_CRITICAL_DIRECTIVE = CursorKind.OMP_CRITICAL_DIRECTIVE¶
- OMP_DISTRIBUTE_DIRECTIVE = CursorKind.OMP_DISTRIBUTE_DIRECTIVE¶
- OMP_DISTRIBUTE_PARALLELFOR_DIRECTIVE = CursorKind.OMP_DISTRIBUTE_PARALLELFOR_DIRECTIVE¶
- OMP_DISTRIBUTE_PARALLEL_FOR_SIMD_DIRECTIVE = CursorKind.OMP_DISTRIBUTE_PARALLEL_FOR_SIMD_DIRECTIVE¶
- OMP_DISTRIBUTE_SIMD_DIRECTIVE = CursorKind.OMP_DISTRIBUTE_SIMD_DIRECTIVE¶
- OMP_FLUSH_DIRECTIVE = CursorKind.OMP_FLUSH_DIRECTIVE¶
- OMP_FOR_DIRECTIVE = CursorKind.OMP_FOR_DIRECTIVE¶
- OMP_FOR_SIMD_DIRECTIVE = CursorKind.OMP_FOR_SIMD_DIRECTIVE¶
- OMP_MASTER_DIRECTIVE = CursorKind.OMP_MASTER_DIRECTIVE¶
- OMP_ORDERED_DIRECTIVE = CursorKind.OMP_ORDERED_DIRECTIVE¶
- OMP_PARALLELFORSIMD_DIRECTIVE = CursorKind.OMP_PARALLELFORSIMD_DIRECTIVE¶
- OMP_PARALLEL_DIRECTIVE = CursorKind.OMP_PARALLEL_DIRECTIVE¶
- OMP_PARALLEL_FOR_DIRECTIVE = CursorKind.OMP_PARALLEL_FOR_DIRECTIVE¶
- OMP_PARALLEL_SECTIONS_DIRECTIVE = CursorKind.OMP_PARALLEL_SECTIONS_DIRECTIVE¶
- OMP_SECTIONS_DIRECTIVE = CursorKind.OMP_SECTIONS_DIRECTIVE¶
- OMP_SECTION_DIRECTIVE = CursorKind.OMP_SECTION_DIRECTIVE¶
- OMP_SIMD_DIRECTIVE = CursorKind.OMP_SIMD_DIRECTIVE¶
- OMP_SINGLE_DIRECTIVE = CursorKind.OMP_SINGLE_DIRECTIVE¶
- OMP_TARGET_DATA_DIRECTIVE = CursorKind.OMP_TARGET_DATA_DIRECTIVE¶
- OMP_TARGET_DIRECTIVE = CursorKind.OMP_TARGET_DIRECTIVE¶
- OMP_TARGET_ENTER_DATA_DIRECTIVE = CursorKind.OMP_TARGET_ENTER_DATA_DIRECTIVE¶
- OMP_TARGET_EXIT_DATA_DIRECTIVE = CursorKind.OMP_TARGET_EXIT_DATA_DIRECTIVE¶
- OMP_TARGET_PARALLELFOR_DIRECTIVE = CursorKind.OMP_TARGET_PARALLELFOR_DIRECTIVE¶
- OMP_TARGET_PARALLEL_DIRECTIVE = CursorKind.OMP_TARGET_PARALLEL_DIRECTIVE¶
- OMP_TARGET_PARALLEL_FOR_SIMD_DIRECTIVE = CursorKind.OMP_TARGET_PARALLEL_FOR_SIMD_DIRECTIVE¶
- OMP_TARGET_SIMD_DIRECTIVE = CursorKind.OMP_TARGET_SIMD_DIRECTIVE¶
- OMP_TARGET_UPDATE_DIRECTIVE = CursorKind.OMP_TARGET_UPDATE_DIRECTIVE¶
- OMP_TASKGROUP_DIRECTIVE = CursorKind.OMP_TASKGROUP_DIRECTIVE¶
- OMP_TASKWAIT_DIRECTIVE = CursorKind.OMP_TASKWAIT_DIRECTIVE¶
- OMP_TASKYIELD_DIRECTIVE = CursorKind.OMP_TASKYIELD_DIRECTIVE¶
- OMP_TASK_DIRECTIVE = CursorKind.OMP_TASK_DIRECTIVE¶
- OMP_TASK_LOOP_DIRECTIVE = CursorKind.OMP_TASK_LOOP_DIRECTIVE¶
- OMP_TASK_LOOP_SIMD_DIRECTIVE = CursorKind.OMP_TASK_LOOP_SIMD_DIRECTIVE¶
- OMP_TEAMS_DIRECTIVE = CursorKind.OMP_TEAMS_DIRECTIVE¶
- OMP_TEAMS_DISTRIBUTE_DIRECTIVE = CursorKind.OMP_TEAMS_DISTRIBUTE_DIRECTIVE¶
- OVERLOADED_DECL_REF = CursorKind.OVERLOADED_DECL_REF¶
- OVERLOAD_CANDIDATE = CursorKind.OVERLOAD_CANDIDATE¶
- PACKED_ATTR = CursorKind.PACKED_ATTR¶
- PACK_EXPANSION_EXPR = CursorKind.PACK_EXPANSION_EXPR¶
- PAREN_EXPR = CursorKind.PAREN_EXPR¶
- PARM_DECL = CursorKind.PARM_DECL¶
- PREPROCESSING_DIRECTIVE = CursorKind.PREPROCESSING_DIRECTIVE¶
- PURE_ATTR = CursorKind.PURE_ATTR¶
- RETURN_STMT = CursorKind.RETURN_STMT¶
- SEH_EXCEPT_STMT = CursorKind.SEH_EXCEPT_STMT¶
- SEH_FINALLY_STMT = CursorKind.SEH_FINALLY_STMT¶
- SEH_LEAVE_STMT = CursorKind.SEH_LEAVE_STMT¶
- SEH_TRY_STMT = CursorKind.SEH_TRY_STMT¶
- SIZE_OF_PACK_EXPR = CursorKind.SIZE_OF_PACK_EXPR¶
- STATIC_ASSERT = CursorKind.STATIC_ASSERT¶
- STRING_LITERAL = CursorKind.STRING_LITERAL¶
- STRUCT_DECL = CursorKind.STRUCT_DECL¶
- SWITCH_STMT = CursorKind.SWITCH_STMT¶
- StmtExpr = CursorKind.StmtExpr¶
- TEMPLATE_NON_TYPE_PARAMETER = CursorKind.TEMPLATE_NON_TYPE_PARAMETER¶
- TEMPLATE_REF = CursorKind.TEMPLATE_REF¶
- TEMPLATE_TEMPLATE_PARAMETER = CursorKind.TEMPLATE_TEMPLATE_PARAMETER¶
- TEMPLATE_TYPE_PARAMETER = CursorKind.TEMPLATE_TYPE_PARAMETER¶
- TRANSLATION_UNIT = CursorKind.TRANSLATION_UNIT¶
- TYPEDEF_DECL = CursorKind.TYPEDEF_DECL¶
- TYPE_ALIAS_DECL = CursorKind.TYPE_ALIAS_DECL¶
- TYPE_ALIAS_TEMPLATE_DECL = CursorKind.TYPE_ALIAS_TEMPLATE_DECL¶
- TYPE_REF = CursorKind.TYPE_REF¶
- UNARY_OPERATOR = CursorKind.UNARY_OPERATOR¶
- UNEXPOSED_ATTR = CursorKind.UNEXPOSED_ATTR¶
- UNEXPOSED_DECL = CursorKind.UNEXPOSED_DECL¶
- UNEXPOSED_EXPR = CursorKind.UNEXPOSED_EXPR¶
- UNEXPOSED_STMT = CursorKind.UNEXPOSED_STMT¶
- UNION_DECL = CursorKind.UNION_DECL¶
- USING_DECLARATION = CursorKind.USING_DECLARATION¶
- USING_DIRECTIVE = CursorKind.USING_DIRECTIVE¶
- VARIABLE_REF = CursorKind.VARIABLE_REF¶
- VAR_DECL = CursorKind.VAR_DECL¶
- VISIBILITY_ATTR = CursorKind.VISIBILITY_ATTR¶
- WARN_UNUSED_ATTR = CursorKind.WARN_UNUSED_ATTR¶
- WARN_UNUSED_RESULT_ATTR = CursorKind.WARN_UNUSED_RESULT_ATTR¶
- WHILE_STMT = CursorKind.WHILE_STMT¶
- class rawtypes.clang.cindex.Cursor¶
ベースクラス:
_ctypes.Structure
The Cursor class represents a reference to an element within the AST. It acts as a kind of iterator.
- static from_location(tu, location)¶
- is_definition()¶
Returns true if the declaration pointed at by the cursor is also a definition of that entity.
- is_const_method()¶
Returns True if the cursor refers to a C++ member function or member function template that is declared 'const'.
- is_converting_constructor()¶
Returns True if the cursor refers to a C++ converting constructor.
- is_copy_constructor()¶
Returns True if the cursor refers to a C++ copy constructor.
- is_default_constructor()¶
Returns True if the cursor refers to a C++ default constructor.
- is_move_constructor()¶
Returns True if the cursor refers to a C++ move constructor.
- is_default_method()¶
Returns True if the cursor refers to a C++ member function or member function template that is declared '= default'.
- is_mutable_field()¶
Returns True if the cursor refers to a C++ field that is declared 'mutable'.
- is_pure_virtual_method()¶
Returns True if the cursor refers to a C++ member function or member function template that is declared pure virtual.
- is_static_method()¶
Returns True if the cursor refers to a C++ member function or member function template that is declared 'static'.
- is_virtual_method()¶
Returns True if the cursor refers to a C++ member function or member function template that is declared 'virtual'.
- is_abstract_record()¶
Returns True if the cursor refers to a C++ record declaration that has pure virtual member functions.
- is_scoped_enum()¶
Returns True if the cursor refers to a scoped enum declaration.
- get_definition()¶
If the cursor is a reference to a declaration or a declaration of some entity, return a cursor that points to the definition of that entity.
- get_usr()¶
Return the Unified Symbol Resolution (USR) for the entity referenced by the given cursor (or None).
A Unified Symbol Resolution (USR) is a string that identifies a particular entity (function, class, variable, etc.) within a program. USRs can be compared across translation units to determine, e.g., when references in one translation refer to an entity defined in another translation unit.
- get_included_file()¶
Returns the File that is included by the current inclusion cursor.
- property kind¶
Return the kind of this cursor.
- property spelling¶
Return the spelling of the entity pointed at by the cursor.
- property displayname¶
Return the display name for the entity referenced by this cursor.
The display name contains extra information that helps identify the cursor, such as the parameters of a function or template or the arguments of a class template specialization.
- property mangled_name¶
Return the mangled name for the entity referenced by this cursor.
- property location¶
Return the source location (the starting character) of the entity pointed at by the cursor.
- property linkage¶
Return the linkage of this cursor.
- property tls_kind¶
Return the thread-local storage (TLS) kind of this cursor.
- property extent¶
Return the source range (the range of text) occupied by the entity pointed at by the cursor.
- property storage_class¶
Retrieves the storage class (if any) of the entity pointed at by the cursor.
- property availability¶
Retrieves the availability of the entity pointed at by the cursor.
- property access_specifier¶
Retrieves the access specifier (if any) of the entity pointed at by the cursor.
- property type¶
Retrieve the Type (if any) of the entity pointed at by the cursor.
- property canonical¶
Return the canonical Cursor corresponding to this Cursor.
The canonical cursor is the cursor which is representative for the underlying entity. For example, if you have multiple forward declarations for the same class, the canonical cursor for the forward declarations will be identical.
- property result_type¶
Retrieve the Type of the result for this Cursor.
- property exception_specification_kind¶
Retrieve the exception specification kind, which is one of the values from the ExceptionSpecificationKind enumeration.
- property underlying_typedef_type¶
Return the underlying type of a typedef declaration.
Returns a Type for the typedef this cursor is a declaration for. If the current cursor is not a typedef, this raises.
- property enum_type¶
Return the integer type of an enum declaration.
Returns a Type corresponding to an integer. If the cursor is not for an enum, this raises.
- property enum_value¶
Return the value of an enum constant.
- property objc_type_encoding¶
Return the Objective-C type encoding as a str.
- property hash¶
Returns a hash of the cursor as an int.
- property semantic_parent¶
Return the semantic parent for this cursor.
- property lexical_parent¶
Return the lexical parent for this cursor.
- property translation_unit¶
Returns the TranslationUnit to which this Cursor belongs.
- property referenced¶
For a cursor that is a reference, returns a cursor representing the entity that it references.
- property brief_comment¶
Returns the brief comment text associated with that Cursor
- property raw_comment¶
Returns the raw comment text associated with that Cursor
- get_arguments()¶
Return an iterator for accessing the arguments of this cursor.
- get_num_template_arguments()¶
Returns the number of template args associated with this cursor.
- get_template_argument_kind(num)¶
Returns the TemplateArgumentKind for the indicated template argument.
- get_template_argument_type(num)¶
Returns the CXType for the indicated template argument.
- get_template_argument_value(num)¶
Returns the value of the indicated arg as a signed 64b integer.
- get_template_argument_unsigned_value(num)¶
Returns the value of the indicated arg as an unsigned 64b integer.
- get_children()¶
Return an iterator for accessing the children of this cursor.
- walk_preorder()¶
Depth-first preorder walk over the cursor and its descendants.
Yields cursors.
- get_tokens()¶
Obtain Token instances formulating that compose this Cursor.
This is a generator for Token instances. It returns all tokens which occupy the extent this cursor occupies.
- get_field_offsetof()¶
Returns the offsetof the FIELD_DECL pointed by this Cursor.
- is_anonymous()¶
Check if the record is anonymous.
- is_bitfield()¶
Check if the field is a bitfield.
- get_bitfield_width()¶
Retrieve the width of a bitfield.
- static from_result(res, fn, args)¶
- static from_cursor_result(res, fn, args)¶
- data¶
Structure/Union member
- xdata¶
Structure/Union member
- class rawtypes.clang.cindex.Diagnostic(ptr)¶
ベースクラス:
object
A Diagnostic is a single instance of a Clang diagnostic. It includes the diagnostic severity, the message, the location the diagnostic occurred, as well as additional source ranges and associated fix-it hints.
- Ignored = 0¶
- Note = 1¶
- Warning = 2¶
- Error = 3¶
- Fatal = 4¶
- DisplaySourceLocation = 1¶
- DisplayColumn = 2¶
- DisplaySourceRanges = 4¶
- DisplayOption = 8¶
- DisplayCategoryId = 16¶
- DisplayCategoryName = 32¶
- property severity¶
- property location¶
- property spelling¶
- property ranges¶
- property fixits¶
- property children¶
- property category_number¶
The category number for this diagnostic or 0 if unavailable.
- property category_name¶
The string name of the category for this diagnostic.
- property option¶
The command-line option that enables this diagnostic.
- property disable_option¶
The command-line option that disables this diagnostic.
- format(options=None)¶
Format this diagnostic for display. The options argument takes Diagnostic.Display* flags, which can be combined using bitwise OR. If the options argument is not provided, the default display options will be used.
- from_param()¶
- class rawtypes.clang.cindex.File(obj)¶
ベースクラス:
rawtypes.clang.cindex.ClangObject
The File class represents a particular source file that is part of a translation unit.
- static from_name(translation_unit, file_name)¶
Retrieve a file handle within the given translation unit.
- property name¶
Return the complete file and path name of the file.
- property time¶
Return the last modification time of the file.
- static from_result(res, fn, args)¶
- class rawtypes.clang.cindex.FixIt(range, value)¶
ベースクラス:
object
A FixIt represents a transformation to be applied to the source to "fix-it". The fix-it shouldbe applied by replacing the given source range with the given value.
- class rawtypes.clang.cindex.Index(obj)¶
ベースクラス:
rawtypes.clang.cindex.ClangObject
The Index type provides the primary interface to the Clang CIndex library, primarily by providing an interface for reading and parsing translation units.
- static create(excludeDecls=False)¶
Create a new Index. Parameters: excludeDecls -- Exclude local declarations from translation units.
- read(path)¶
Load a TranslationUnit from the given AST file.
- parse(path, args=None, unsaved_files=None, options=0)¶
Load the translation unit from the given source code file by running clang and generating the AST before loading. Additional command line parameters can be passed to clang via the args parameter.
In-memory contents for files can be provided by passing a list of pairs to as unsaved_files, the first item should be the filenames to be mapped and the second should be the contents to be substituted for the file. The contents may be passed as strings or file objects.
If an error was encountered during parsing, a TranslationUnitLoadError will be raised.
- class rawtypes.clang.cindex.LinkageKind(value)¶
ベースクラス:
rawtypes.clang.cindex.BaseEnumeration
Describes the kind of linkage of a cursor.
- from_param()¶
- EXTERNAL = LinkageKind.EXTERNAL¶
- INTERNAL = LinkageKind.INTERNAL¶
- INVALID = LinkageKind.INVALID¶
- NO_LINKAGE = LinkageKind.NO_LINKAGE¶
- UNIQUE_EXTERNAL = LinkageKind.UNIQUE_EXTERNAL¶
- class rawtypes.clang.cindex.SourceLocation¶
ベースクラス:
_ctypes.Structure
A SourceLocation represents a particular location within a source file.
- static from_position(tu, file, line, column)¶
Retrieve the source location associated with a given file/line/column in a particular translation unit.
- static from_offset(tu, file, offset)¶
Retrieve a SourceLocation from a given character offset.
tu -- TranslationUnit file belongs to file -- File instance to obtain offset from offset -- Integer character offset within file
- property file¶
Get the file represented by this source location.
- property line¶
Get the line represented by this source location.
- property column¶
Get the column represented by this source location.
- property offset¶
Get the file offset represented by this source location.
- int_data¶
Structure/Union member
- ptr_data¶
Structure/Union member
- class rawtypes.clang.cindex.SourceRange¶
ベースクラス:
_ctypes.Structure
A SourceRange describes a range of source locations within the source code.
- static from_locations(start, end)¶
- property start¶
Return a SourceLocation representing the first character within a source range.
- property end¶
Return a SourceLocation representing the last character within a source range.
- begin_int_data¶
Structure/Union member
- end_int_data¶
Structure/Union member
- ptr_data¶
Structure/Union member
- class rawtypes.clang.cindex.TLSKind(value)¶
ベースクラス:
rawtypes.clang.cindex.BaseEnumeration
Describes the kind of thread-local storage (TLS) of a cursor.
- from_param()¶
- DYNAMIC = TLSKind.DYNAMIC¶
- NONE = TLSKind.NONE¶
- STATIC = TLSKind.STATIC¶
- class rawtypes.clang.cindex.TokenKind(value, name)¶
ベースクラス:
object
Describes a specific type of a Token.
- static from_value(value)¶
Obtain a registered TokenKind instance from its value.
- static register(value, name)¶
Register a new TokenKind enumeration.
This should only be called at module load time by code within this package.
- COMMENT = TokenKind.COMMENT¶
- IDENTIFIER = TokenKind.IDENTIFIER¶
- KEYWORD = TokenKind.KEYWORD¶
- LITERAL = TokenKind.LITERAL¶
- PUNCTUATION = TokenKind.PUNCTUATION¶
- class rawtypes.clang.cindex.Token¶
ベースクラス:
_ctypes.Structure
Represents a single token from the preprocessor.
Tokens are effectively segments of source code. Source code is first parsed into tokens before being converted into the AST and Cursors.
Tokens are obtained from parsed TranslationUnit instances. You currently can't create tokens manually.
- property spelling¶
The spelling of this token.
This is the textual representation of the token in source.
- property kind¶
Obtain the TokenKind of the current token.
- property location¶
The SourceLocation this Token occurs at.
- property extent¶
The SourceRange this Token occupies.
- property cursor¶
The Cursor this Token corresponds to.
- int_data¶
Structure/Union member
- ptr_data¶
Structure/Union member
- exception rawtypes.clang.cindex.TranslationUnitLoadError¶
ベースクラス:
Exception
Represents an error that occurred when loading a TranslationUnit.
This is raised in the case where a TranslationUnit could not be instantiated due to failure in the libclang library.
FIXME: Make libclang expose additional error information in this scenario.
- class rawtypes.clang.cindex.TranslationUnit(ptr, index)¶
ベースクラス:
rawtypes.clang.cindex.ClangObject
Represents a source code translation unit.
This is one of the main types in the API. Any time you wish to interact with Clang's representation of a source file, you typically start with a translation unit.
- PARSE_NONE = 0¶
- PARSE_DETAILED_PROCESSING_RECORD = 1¶
- PARSE_INCOMPLETE = 2¶
- PARSE_PRECOMPILED_PREAMBLE = 4¶
- PARSE_CACHE_COMPLETION_RESULTS = 8¶
- PARSE_SKIP_FUNCTION_BODIES = 64¶
- PARSE_INCLUDE_BRIEF_COMMENTS_IN_CODE_COMPLETION = 128¶
- classmethod from_source(filename, args=None, unsaved_files=None, options=0, index=None)¶
Create a TranslationUnit by parsing source.
This is capable of processing source code both from files on the filesystem as well as in-memory contents.
Command-line arguments that would be passed to clang are specified as a list via args. These can be used to specify include paths, warnings, etc. e.g. ["-Wall", "-I/path/to/include"].
In-memory file content can be provided via unsaved_files. This is an iterable of 2-tuples. The first element is the filename (str or PathLike). The second element defines the content. Content can be provided as str source code or as file objects (anything with a read() method). If a file object is being used, content will be read until EOF and the read cursor will not be reset to its original position.
options is a bitwise or of TranslationUnit.PARSE_XXX flags which will control parsing behavior.
index is an Index instance to utilize. If not provided, a new Index will be created for this TranslationUnit.
To parse source from the filesystem, the filename of the file to parse is specified by the filename argument. Or, filename could be None and the args list would contain the filename(s) to parse.
To parse source from an in-memory buffer, set filename to the virtual filename you wish to associate with this source (e.g. "test.c"). The contents of that file are then provided in unsaved_files.
If an error occurs, a TranslationUnitLoadError is raised.
Please note that a TranslationUnit with parser errors may be returned. It is the caller's responsibility to check tu.diagnostics for errors.
Also note that Clang infers the source language from the extension of the input filename. If you pass in source code containing a C++ class declaration with the filename "test.c" parsing will fail.
- classmethod from_ast_file(filename, index=None)¶
Create a TranslationUnit instance from a saved AST file.
A previously-saved AST file (provided with -emit-ast or TranslationUnit.save()) is loaded from the filename specified.
If the file cannot be loaded, a TranslationUnitLoadError will be raised.
index is optional and is the Index instance to use. If not provided, a default Index will be created.
filename can be str or PathLike.
- property cursor¶
Retrieve the cursor that represents the given translation unit.
- property spelling¶
Get the original translation unit source file name.
- get_includes()¶
Return an iterable sequence of FileInclusion objects that describe the sequence of inclusions in a translation unit. The first object in this sequence is always the input file. Note that this method will not recursively iterate over header files included through precompiled headers.
- get_file(filename)¶
Obtain a File from this translation unit.
- get_location(filename, position)¶
Obtain a SourceLocation for a file in this translation unit.
The position can be specified by passing:
Integer file offset. Initial file offset is 0.
2-tuple of (line number, column number). Initial file position is (0, 0)
- get_extent(filename, locations)¶
Obtain a SourceRange from this translation unit.
The bounds of the SourceRange must ultimately be defined by a start and end SourceLocation. For the locations argument, you can pass:
2 SourceLocation instances in a 2-tuple or list.
2 int file offsets via a 2-tuple or list.
2 2-tuple or lists of (line, column) pairs in a 2-tuple or list.
e.g.
get_extent('foo.c', (5, 10)) get_extent('foo.c', ((1, 1), (1, 15)))
- property diagnostics¶
Return an iterable (and indexable) object containing the diagnostics.
- reparse(unsaved_files=None, options=0)¶
Reparse an already parsed translation unit.
In-memory contents for files can be provided by passing a list of pairs as unsaved_files, the first items should be the filenames to be mapped and the second should be the contents to be substituted for the file. The contents may be passed as strings or file objects.
- save(filename)¶
Saves the TranslationUnit to a file.
This is equivalent to passing -emit-ast to the clang frontend. The saved file can be loaded back into a TranslationUnit. Or, if it corresponds to a header, it can be used as a pre-compiled header file.
If an error occurs while saving, a TranslationUnitSaveError is raised. If the error was TranslationUnitSaveError.ERROR_INVALID_TU, this means the constructed TranslationUnit was not valid at time of save. In this case, the reason(s) why should be available via TranslationUnit.diagnostics().
filename -- The path to save the translation unit to (str or PathLike).
- codeComplete(path, line, column, unsaved_files=None, include_macros=False, include_code_patterns=False, include_brief_comments=False)¶
Code complete in this translation unit.
In-memory contents for files can be provided by passing a list of pairs as unsaved_files, the first items should be the filenames to be mapped and the second should be the contents to be substituted for the file. The contents may be passed as strings or file objects.
- get_tokens(locations=None, extent=None)¶
Obtain tokens in this translation unit.
This is a generator for Token instances. The caller specifies a range of source code to obtain tokens for. The range can be specified as a 2-tuple of SourceLocation or as a SourceRange. If both are defined, behavior is undefined.
- class rawtypes.clang.cindex.TypeKind(value)¶
ベースクラス:
rawtypes.clang.cindex.BaseEnumeration
Describes the kind of type.
- property spelling¶
Retrieve the spelling of this TypeKind.
- ATOMIC = TypeKind.ATOMIC¶
- AUTO = TypeKind.AUTO¶
- BLOCKPOINTER = TypeKind.BLOCKPOINTER¶
- BOOL = TypeKind.BOOL¶
- CHAR16 = TypeKind.CHAR16¶
- CHAR32 = TypeKind.CHAR32¶
- CHAR_S = TypeKind.CHAR_S¶
- CHAR_U = TypeKind.CHAR_U¶
- COMPLEX = TypeKind.COMPLEX¶
- CONSTANTARRAY = TypeKind.CONSTANTARRAY¶
- DEPENDENT = TypeKind.DEPENDENT¶
- DEPENDENTSIZEDARRAY = TypeKind.DEPENDENTSIZEDARRAY¶
- DOUBLE = TypeKind.DOUBLE¶
- ELABORATED = TypeKind.ELABORATED¶
- ENUM = TypeKind.ENUM¶
- EXTVECTOR = TypeKind.EXTVECTOR¶
- FLOAT = TypeKind.FLOAT¶
- FLOAT128 = TypeKind.FLOAT128¶
- FUNCTIONNOPROTO = TypeKind.FUNCTIONNOPROTO¶
- FUNCTIONPROTO = TypeKind.FUNCTIONPROTO¶
- HALF = TypeKind.HALF¶
- INCOMPLETEARRAY = TypeKind.INCOMPLETEARRAY¶
- INT = TypeKind.INT¶
- INT128 = TypeKind.INT128¶
- INVALID = TypeKind.INVALID¶
- LONG = TypeKind.LONG¶
- LONGDOUBLE = TypeKind.LONGDOUBLE¶
- LONGLONG = TypeKind.LONGLONG¶
- LVALUEREFERENCE = TypeKind.LVALUEREFERENCE¶
- MEMBERPOINTER = TypeKind.MEMBERPOINTER¶
- NULLPTR = TypeKind.NULLPTR¶
- OBJCCLASS = TypeKind.OBJCCLASS¶
- OBJCID = TypeKind.OBJCID¶
- OBJCINTERFACE = TypeKind.OBJCINTERFACE¶
- OBJCOBJECTPOINTER = TypeKind.OBJCOBJECTPOINTER¶
- OBJCSEL = TypeKind.OBJCSEL¶
- OCLEVENT = TypeKind.OCLEVENT¶
- OCLIMAGE1DARRAYRO = TypeKind.OCLIMAGE1DARRAYRO¶
- OCLIMAGE1DARRAYRW = TypeKind.OCLIMAGE1DARRAYRW¶
- OCLIMAGE1DARRAYWO = TypeKind.OCLIMAGE1DARRAYWO¶
- OCLIMAGE1DBUFFERRO = TypeKind.OCLIMAGE1DBUFFERRO¶
- OCLIMAGE1DBUFFERRW = TypeKind.OCLIMAGE1DBUFFERRW¶
- OCLIMAGE1DBUFFERWO = TypeKind.OCLIMAGE1DBUFFERWO¶
- OCLIMAGE1DRO = TypeKind.OCLIMAGE1DRO¶
- OCLIMAGE1DRW = TypeKind.OCLIMAGE1DRW¶
- OCLIMAGE1DWO = TypeKind.OCLIMAGE1DWO¶
- OCLIMAGE2DARRAYDEPTHRO = TypeKind.OCLIMAGE2DARRAYDEPTHRO¶
- OCLIMAGE2DARRAYDEPTHRW = TypeKind.OCLIMAGE2DARRAYDEPTHRW¶
- OCLIMAGE2DARRAYDEPTHWO = TypeKind.OCLIMAGE2DARRAYDEPTHWO¶
- OCLIMAGE2DARRAYMSAADEPTHRO = TypeKind.OCLIMAGE2DARRAYMSAADEPTHRO¶
- OCLIMAGE2DARRAYMSAADEPTHRW = TypeKind.OCLIMAGE2DARRAYMSAADEPTHRW¶
- OCLIMAGE2DARRAYMSAADEPTHWO = TypeKind.OCLIMAGE2DARRAYMSAADEPTHWO¶
- OCLIMAGE2DARRAYMSAARO = TypeKind.OCLIMAGE2DARRAYMSAARO¶
- OCLIMAGE2DARRAYMSAARW = TypeKind.OCLIMAGE2DARRAYMSAARW¶
- OCLIMAGE2DARRAYMSAAWO = TypeKind.OCLIMAGE2DARRAYMSAAWO¶
- OCLIMAGE2DARRAYRO = TypeKind.OCLIMAGE2DARRAYRO¶
- OCLIMAGE2DARRAYRW = TypeKind.OCLIMAGE2DARRAYRW¶
- OCLIMAGE2DARRAYWO = TypeKind.OCLIMAGE2DARRAYWO¶
- OCLIMAGE2DDEPTHRO = TypeKind.OCLIMAGE2DDEPTHRO¶
- OCLIMAGE2DDEPTHRW = TypeKind.OCLIMAGE2DDEPTHRW¶
- OCLIMAGE2DDEPTHWO = TypeKind.OCLIMAGE2DDEPTHWO¶
- OCLIMAGE2DMSAADEPTHRO = TypeKind.OCLIMAGE2DMSAADEPTHRO¶
- OCLIMAGE2DMSAADEPTHRW = TypeKind.OCLIMAGE2DMSAADEPTHRW¶
- OCLIMAGE2DMSAADEPTHWO = TypeKind.OCLIMAGE2DMSAADEPTHWO¶
- OCLIMAGE2DMSAARO = TypeKind.OCLIMAGE2DMSAARO¶
- OCLIMAGE2DMSAARW = TypeKind.OCLIMAGE2DMSAARW¶
- OCLIMAGE2DMSAAWO = TypeKind.OCLIMAGE2DMSAAWO¶
- OCLIMAGE2DRO = TypeKind.OCLIMAGE2DRO¶
- OCLIMAGE2DRW = TypeKind.OCLIMAGE2DRW¶
- OCLIMAGE2DWO = TypeKind.OCLIMAGE2DWO¶
- OCLIMAGE3DRO = TypeKind.OCLIMAGE3DRO¶
- OCLIMAGE3DRW = TypeKind.OCLIMAGE3DRW¶
- OCLIMAGE3DWO = TypeKind.OCLIMAGE3DWO¶
- OCLQUEUE = TypeKind.OCLQUEUE¶
- OCLRESERVEID = TypeKind.OCLRESERVEID¶
- OCLSAMPLER = TypeKind.OCLSAMPLER¶
- OVERLOAD = TypeKind.OVERLOAD¶
- PIPE = TypeKind.PIPE¶
- POINTER = TypeKind.POINTER¶
- RECORD = TypeKind.RECORD¶
- RVALUEREFERENCE = TypeKind.RVALUEREFERENCE¶
- SCHAR = TypeKind.SCHAR¶
- SHORT = TypeKind.SHORT¶
- TYPEDEF = TypeKind.TYPEDEF¶
- UCHAR = TypeKind.UCHAR¶
- UINT = TypeKind.UINT¶
- UINT128 = TypeKind.UINT128¶
- ULONG = TypeKind.ULONG¶
- ULONGLONG = TypeKind.ULONGLONG¶
- UNEXPOSED = TypeKind.UNEXPOSED¶
- USHORT = TypeKind.USHORT¶
- VARIABLEARRAY = TypeKind.VARIABLEARRAY¶
- VECTOR = TypeKind.VECTOR¶
- VOID = TypeKind.VOID¶
- WCHAR = TypeKind.WCHAR¶
- class rawtypes.clang.cindex.Type¶
ベースクラス:
_ctypes.Structure
The type of an element in the abstract syntax tree.
- property kind¶
Return the kind of this type.
- argument_types()¶
Retrieve a container for the non-variadic arguments for this type.
The returned object is iterable and indexable. Each item in the container is a Type instance.
- property element_type¶
Retrieve the Type of elements within this Type.
If accessed on a type that is not an array, complex, or vector type, an exception will be raised.
- property element_count¶
Retrieve the number of elements in this type.
Returns an int.
If the Type is not an array or vector, this raises.
- property translation_unit¶
The TranslationUnit to which this Type is associated.
- static from_result(res, fn, args)¶
- get_num_template_arguments()¶
- get_template_argument_type(num)¶
- get_canonical()¶
Return the canonical type for a Type.
Clang's type system explicitly models typedefs and all the ways a specific type can be represented. The canonical type is the underlying type with all the "sugar" removed. For example, if 'T' is a typedef for 'int', the canonical type for 'T' would be 'int'.
- is_const_qualified()¶
Determine whether a Type has the "const" qualifier set.
This does not look through typedefs that may have added "const" at a different level.
- is_volatile_qualified()¶
Determine whether a Type has the "volatile" qualifier set.
This does not look through typedefs that may have added "volatile" at a different level.
- is_restrict_qualified()¶
Determine whether a Type has the "restrict" qualifier set.
This does not look through typedefs that may have added "restrict" at a different level.
- is_function_variadic()¶
Determine whether this function Type is a variadic function type.
- get_address_space()¶
- get_typedef_name()¶
- is_pod()¶
Determine whether this Type represents plain old data (POD).
- get_pointee()¶
For pointer types, returns the type of the pointee.
- get_declaration()¶
Return the cursor for the declaration of the given type.
- get_result()¶
Retrieve the result type associated with a function type.
- get_array_element_type()¶
Retrieve the type of the elements of the array type.
- get_array_size()¶
Retrieve the size of the constant array.
- get_class_type()¶
Retrieve the class type of the member pointer type.
- get_named_type()¶
Retrieve the type named by the qualified-id.
- get_align()¶
Retrieve the alignment of the record.
- get_size()¶
Retrieve the size of the record.
- get_offset(fieldname)¶
Retrieve the offset of a field in the record.
- get_ref_qualifier()¶
Retrieve the ref-qualifier of the type.
- get_fields()¶
Return an iterator for accessing the fields of this type.
- get_exception_specification_kind()¶
Return the kind of the exception specification; a value from the ExceptionSpecificationKind enumeration.
- property spelling¶
Retrieve the spelling of this Type.
- data¶
Structure/Union member